This article is currently in the process of being translated into Persian (~42% done).
The TextBox control
کنترل جعبه ی متن ساده ترین کنترل ورودی متن موجود در WPF میباشد که به کاربر اجازه ی وارد کردن متن ساده به صورت یک خطی برای جواب دهی به دیالوگ ها و یا چند خطی مانند ویرایشگر های متن را می دهد
جعبه ی متن یک خطی
کنترل TextBox یک چیز معمولی است که در واقع نیازی به استفاده از هیچ ویژگی روی آن ندارید تا یک فیلد متنی قابل ویرایش کامل داشته باشید. در اینجا یک مثال آشکار آورده شده است:
<Window x:Class="WpfTutorialSamples.Basic_controls.TextBoxSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="TextBoxSample" Height="80" Width="250">
<StackPanel Margin="10">
<TextBox />
</StackPanel>
</Window>
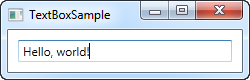
این تمام چیزی است که برای دریافت یک فیلد متنی نیاز دارید. من متن را پس از اجرای نمونه و قبل از گرفتن اسکرین شات اضافه کردم، اما میتوانید این کار را از طریق نشانهگذاری نیز انجام دهید، تا با استفاده از ویژگی Text، جعبه متن را از قبل پر کنید:
<TextBox Text="Hello, world!" />
سعی کنید در TextBox کلیک راست کنید. منویی از گزینه ها را دریافت خواهید کرد که به شما امکان می دهد از TextBox با کلیپ بورد ویندوز استفاده کنید. میانبرهای پیشفرض صفحهکلید برای لغو و انجام مجدد (Ctrl+Z و Ctrl+Y) نیز باید کار کنند، و همه این قابلیتها را رایگان دریافت میکنید!
Multi-line TextBox
اگر مثال بالا را اجرا کنید، متوجه می شوید که کنترل TextBox به طور پیش فرض یک کنترل تک خطی است. وقتی Enter را فشار میدهید، هیچ اتفاقی نمیافتد و اگر متنی بیشتر از آنچه در یک خط قرار میگیرد اضافه کنید، کنترل فقط اسکرول میشود. با این حال، تبدیل کنترل TextBox به یک ویرایشگر چند خطی بسیار ساده است:
<Window x:Class="WpfTutorialSamples.Basic_controls.TextBoxSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="TextBoxSample" Height="160" Width="280">
<Grid Margin="10">
<TextBox AcceptsReturn="True" TextWrapping="Wrap" />
</Grid>
</Window>
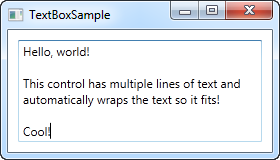
من دو ویژگی اضافه کردهام: AcceptsReturn با اجازه دادن به استفاده از کلید Enter/Return برای رفتن به خط بعدی، TextBox را به یک کنترل چند خطی تبدیل میکند، و ویژگی TextWrapping، که باعث میشود متن بهطور خودکار پس از پایان خط بسته شود. به یک خط رسیده است
Spellcheck with TextBox
As an added bonus, the TextBox control actually comes with automatic spell checking for English and a couple of other languages (as of writing, English, French, German, and Spanish languages are supported).
It works much like in Microsoft Word, where spelling errors are underlined and you can right-click it for suggested alternatives. Enabling spell checking is very easy:
<Window x:Class="WpfTutorialSamples.Basic_controls.TextBoxSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="TextBoxSample" Height="160" Width="280">
<Grid Margin="10">
<TextBox AcceptsReturn="True" TextWrapping="Wrap" SpellCheck.IsEnabled="True" Language="en-US" />
</Grid>
</Window>
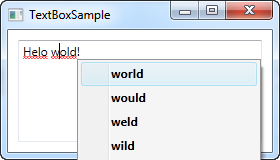
We have used the previous, multi-line textbox example as the basis and then I have added two new properties: The attached property from the SpellCheck class called IsEnabled, which simply enables spell checking on the parent control, and the Language property, which instructs the spell checker which language to use.
Working with TextBox selections
Just like any other editable control in Windows, the TextBox allows for selection of text, e.g. to delete an entire word at once or to copy a piece of the text to the clipboard. The WPF TextBox has several properties for working with selected text, all of them which you can read or even modify. In the next example, we will be reading these properties:
<Window x:Class="WpfTutorialSamples.Basic_controls.TextBoxSelectionSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="TextBoxSelectionSample" Height="150" Width="300">
<DockPanel Margin="10">
<TextBox SelectionChanged="TextBox_SelectionChanged" DockPanel.Dock="Top" />
<TextBox Name="txtStatus" AcceptsReturn="True" TextWrapping="Wrap" IsReadOnly="True" />
</DockPanel>
</Window>
The example consists of two TextBox controls: One for editing and one for outputting the current selection status to. For this, we set the IsReadOnly property to true, to prevent editing of the status TextBox. We subscribe the SelectionChanged event on the first TextBox, which we handle in the Code-behind:
using System;
using System.Text;
using System.Windows;
using System.Windows.Controls;
namespace WpfTutorialSamples.Basic_controls
{
public partial class TextBoxSelectionSample : Window
{
public TextBoxSelectionSample()
{
InitializeComponent();
}
private void TextBox_SelectionChanged(object sender, RoutedEventArgs e)
{
TextBox textBox = sender as TextBox;
txtStatus.Text = "Selection starts at character #" + textBox.SelectionStart + Environment.NewLine;
txtStatus.Text += "Selection is " + textBox.SelectionLength + " character(s) long" + Environment.NewLine;
txtStatus.Text += "Selected text: '" + textBox.SelectedText + "'";
}
}
}
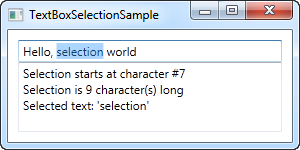
We use three interesting properties to accomplish this:
SelectionStart , which gives us the current cursor position or if there's a selection: Where it starts.
SelectionLength , which gives us the length of the current selection, if any. Otherwise it will just return 0.
SelectedText , which gives us the currently selected string if there's a selection. Otherwise an empty string is returned.
Modifying the selection
All of these properties are both readable and writable, which means that you can modify them as well. For instance, you can set the SelectionStart and SelectionLength properties to select a custom range of text, or you can use the SelectedText property to insert and select a string. Just remember that the TextBox has to have focus, e.g. by calling the Focus() method first, for this to work.