The community is working on translating this tutorial into Serbian, but it seems that no one has started the translation process for this article yet. If you can help us, then please click "More info".
The WindowsFormsHost control
WPF and WinForms are two distinct UI frameworks, both created by Microsoft. WPF is meant as a more modern alternative to WinForms, which was the first .NET UI framework. To lighten the transition between the two, Microsoft has made sure that WinForms controls may still be used inside of a WPF application. This is done with the WindowsFormsHost, which we'll discuss in this article.
To use the WindowsFormsHost and controls from WinForms, you need to add a reference to the following assemblies in your application:
- WindowsFormsIntegration
- System.Windows.Forms
In Visual Studio, this is done by right-clicking the "References" node in your project and selecting "Add reference":
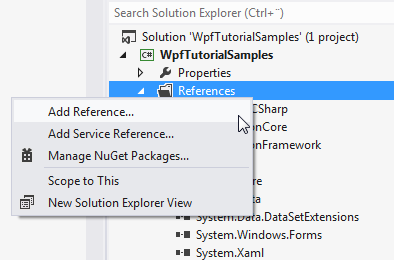
In the dialog that pops up, you should select "Assemblies" and then check the two assemblies that we need to add:
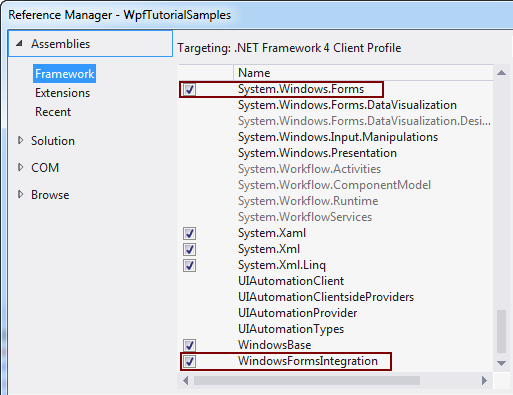
Using the WinForms WebBrowser control
In a previous article, we used the WPF WebBrowser control to create a small web browser. However, as stated in that article, the WPF WebBrowser control is a bit limited when compared to the WinForms version. There are many examples on things easily done with the WinForms version, which are either harder or impossible to do with the WPF version.
A small example is the DocumentTitle property and corresponding DocumentTitleChanged event, which makes it easy to get and update the title of the window to match the title of the current webpage. We'll use this as an excuse to test out the WinForms version right here in our WPF application:
<Window x:Class="WpfTutorialSamples.Misc_controls.WindowsFormsHostSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:wf="clr-namespace:System.Windows.Forms;assembly=System.Windows.Forms"
Title="WindowsFormsHostSample" Height="350" Width="450">
<Grid>
<WindowsFormsHost Name="wfhSample">
<WindowsFormsHost.Child>
<wf:WebBrowser DocumentTitleChanged="wbWinForms_DocumentTitleChanged" />
</WindowsFormsHost.Child>
</WindowsFormsHost>
</Grid>
</Window>
using System;
using System.Windows;
namespace WpfTutorialSamples.Misc_controls
{
public partial class WindowsFormsHostSample : Window
{
public WindowsFormsHostSample()
{
InitializeComponent();
(wfhSample.Child as System.Windows.Forms.WebBrowser).Navigate("http://www.wpf-tutorial.com");
}
private void wbWinForms_DocumentTitleChanged(object sender, EventArgs e)
{
this.Title = (sender as System.Windows.Forms.WebBrowser).DocumentTitle;
}
}
}
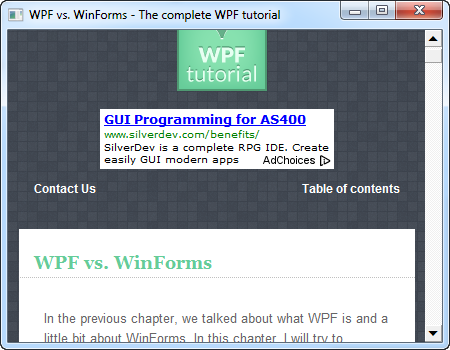
Pay special attention to the line where we add the WinForms namespace to the window, so that we may reference controls from it:
xmlns:wf="clr-namespace:System.Windows.Forms;assembly=System.Windows.Forms"
This will allow us to reference WinForms controls using the wf: prefix.
The WindowsFormsHost is fairly simple to use, as you can see. It has a Child property, in which you can define a single WinForms control, much like the WPF Window only holds a single root control. If you need more controls from WinForms inside of your WindowsFormsHost, you can use the Panel control from WinForms or any of the other container controls.
The WinForms WebBrowser control is used by referencing the System.Windows.Forms assembly, using the wf prefix, as explained above.
In Code-behind, we do an initial call to Navigate, to have a visible webpage instead of the empty control on startup. We then handle theDocumentTitleChanged event, in which we update the Title property of the Window in accordance with the current DocumentTitle value of the WebBrowser control.
Congratulations, you now have a WPF application with a WinForms WebBrowser hosted inside of it.
Summary
As you can see, using WinForms controls inside of your WPF applications is pretty easy, but the question remains: Is it a good idea?
In general, you may want to avoid it. There are a number of issues that may or may not affect your application (a lot of them are described in this MSDN article: http://msdn.microsoft.com/en-us/library/aa970911%28v=VS.100%29.aspx), but a more serious problem is that this kind of UI framework mixing might not be supported in future versions of the .NET framework.
In the end though, the decision is up to you - do you really need the WinForms control or is there a WPF alternative that might work just as well?