The community is working on translating this tutorial into Serbian, but it seems that no one has started the translation process for this article yet. If you can help us, then please click "More info".
DataGrid columns
In the previous chapter, we had a look at just how easy you could get a WPF DataGrid up and running. One of the reasons why it was so easy is the fact that the DataGrid will automatically generate appropriate columns for you, based on the data source you use.
However, in some situations you might want to manually define the columns shown, either because you don’t want all the properties/columns of the data source, or because you want to be in control of which inline editors are used.
Manually defined columns
Let's try an example that looks a lot like the one in the previous chapter, but where we define all the columns manually, for maximum control. You can select the column type based on the data that you wish to display/edit. As of writing, the following column types are available:
- DataGridTextColumn
- DataGridCheckBoxColumn
- DataGridComboBoxColumn
- DataGridHyperlinkColumn
- DataGridTemplateColumn
Especially the last one, the DataGridTemplateColumn, is interesting. It allows you to define any kind of content, which opens up the opportunity to use custom controls, either from the WPF library or even your own or 3rd party controls. Here's an example:
<Window x:Class="WpfTutorialSamples.DataGrid_control.DataGridColumnsSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="DataGridColumnsSample" Height="200" Width="300">
<Grid Margin="10">
<DataGrid Name="dgUsers" AutoGenerateColumns="False">
<DataGrid.Columns>
<DataGridTextColumn Header="Name" Binding="{Binding Name}" />
<DataGridTemplateColumn Header="Birthday">
<DataGridTemplateColumn.CellTemplate>
<DataTemplate>
<DatePicker SelectedDate="{Binding Birthday}" BorderThickness="0" />
</DataTemplate>
</DataGridTemplateColumn.CellTemplate>
</DataGridTemplateColumn>
</DataGrid.Columns>
</DataGrid>
</Grid>
</Window>
using System;
using System.Collections.Generic;
using System.Windows;
namespace WpfTutorialSamples.DataGrid_control
{
public partial class DataGridColumnsSample : Window
{
public DataGridColumnsSample()
{
InitializeComponent();
List<User> users = new List<User>();
users.Add(new User() { Id = 1, Name = "John Doe", Birthday = new DateTime(1971, 7, 23) });
users.Add(new User() { Id = 2, Name = "Jane Doe", Birthday = new DateTime(1974, 1, 17) });
users.Add(new User() { Id = 3, Name = "Sammy Doe", Birthday = new DateTime(1991, 9, 2) });
dgUsers.ItemsSource = users;
}
}
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public DateTime Birthday { get; set; }
}
}
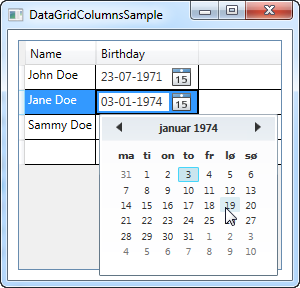
In the markup, I have added the AutoGenerateColumns property on the DataGrid, which I have set to false, to get control of the columns used. As you can see, I have left out the ID column, as I decided that I didn't care for it for this example. For the Name property, I've used a simple text based column, so the most interesting part of this example comes with the Birthday column, where I've used a DataGridTemplateColumn with a DatePicker control inside of it. This allows the end-user to pick the date from a calendar, instead of having to manually enter it, as you can see on the screenshot.
Summary
By turning off automatically generated columns using the AutoGenerateColumns property, you get full control of which columns are shown and how their data should be viewed and edited. As seen by the example of this article, this opens up for some pretty interesting possibilities, where you can completely customize the editor and thereby enhance the end-user experience.