This article has been localized into Korean by the community.
WPF의 예외 처리
C# 또는 그 외 WPF 등 .NET 언어에 익숙하다면 예외 처리가 새롭게 느껴지지 않을 것입니다 : 예외를 던질 수 있는 코드가 있을 때마다 try-catch 블록으로 감싸서 우아하게 예외를 처리해야 합니다. 예를 들어 다음을 고려해봅시다 :
private void Button_Click(object sender, RoutedEventArgs e)
{
string s = null;
s.Trim();
}
이는 명백하게 이상이 있을 것입니다. Trim() 메소드를 현재 null인 변수에 적용했기 때문입니다. 만약 예외처리를 하지 않으면 어플리케이션이 충돌할 것이며 Windows가 그 문제를 다룰 것입니다. 다음에서 볼 수 있듯이 사용자 친화적이지 않습니다 :
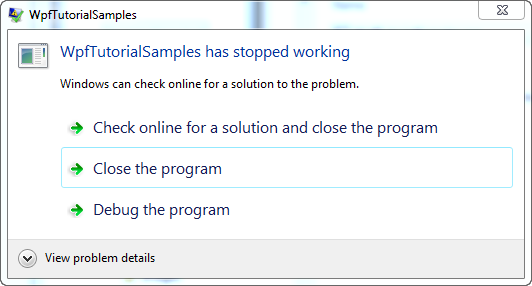
이 경우 쉽게 피할 수 있었던 간단한 에러로 인해 사용자는 강제로 어플리케이션을 종료해야 합니다. 만약 무언가 잘못될 수 있다는 것을 알고 있다면 다음과 같이 try-catch를 사용해야 합니다.
private void Button_Click(object sender, RoutedEventArgs e)
{
string s = null;
try
{
s.Trim();
}
catch(Exception ex)
{
MessageBox.Show("A handled exception just occurred: " + ex.Message, "Exception Sample", MessageBoxButton.OK, MessageBoxImage.Warning);
}
}
하지만 때때로 매우 간단한 코드에서도 예외를 던질 수 있습니다. 이때 try-catch로 코드의 각 줄을 감싸는 방법이 있지만 WPF에서는 전역으로 예외 처리가 가능합니다. Application 클래스의 DispatcherUnhandledException 이벤트를 통해서 말입니다. 이 이벤트를 구독하면, 코드 내에서 미처 처리하지 못한 예외가 던져졌을 때 WPF가 메소드를 호출할 것입니다. 다음은 우리가 위에서 겪었던 상황을 기반으로 한 예제입니다.
<Window x:Class="WpfTutorialSamples.WPF_Application.ExceptionHandlingSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="ExceptionHandlingSample" Height="200" Width="200">
<Grid>
<Button HorizontalAlignment="Center" VerticalAlignment="Center" Click="Button_Click">
Do something bad!
</Button>
</Grid>
</Window>
using System;
using System.Windows;
namespace WpfTutorialSamples.WPF_Application
{
public partial class ExceptionHandlingSample : Window
{
public ExceptionHandlingSample()
{
InitializeComponent();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
string s = null;
try
{
s.Trim();
}
catch(Exception ex)
{
MessageBox.Show("A handled exception just occurred: " + ex.Message, "Exception Sample", MessageBoxButton.OK, MessageBoxImage.Warning);
}
s.Trim();
}
}
}
try-catch 블록 밖에 Trim() 메소드를 한번 더 호출했습니다. 즉 첫 번째 호출은 처리되었지만 두 번째 호출은 처리되지 않았습니다. 두 번째 경우를 위해서 우리는 App.xaml 마술이 필요합니다 :
<Application x:Class="WpfTutorialSamples.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
DispatcherUnhandledException="Application_DispatcherUnhandledException"
StartupUri="WPF Application/ExceptionHandlingSample.xaml">
<Application.Resources>
</Application.Resources>
</Application>
using System;
using System.Windows;
namespace WpfTutorialSamples
{
public partial class App : Application
{
private void Application_DispatcherUnhandledException(object sender, System.Windows.Threading.DispatcherUnhandledExceptionEventArgs e)
{
MessageBox.Show("An unhandled exception just occurred: " + e.Exception.Message, "Exception Sample", MessageBoxButton.OK, MessageBoxImage.Error);
e.Handled = true;
}
}
}
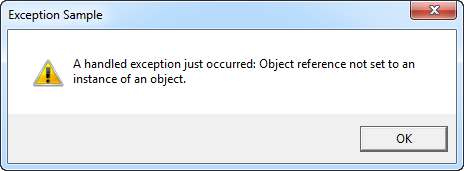
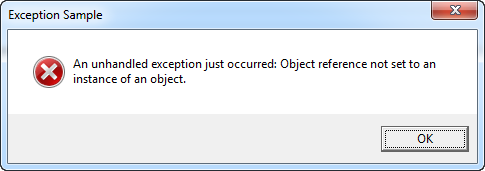
로컬에서와 마찬가지로 예외를 처리합니다. 하지만 메시지 박스의 텍스트와 이미지가 아주 약간 다릅니다. 또한 e.Handled 속성을 true로 설정했습니다. 이 속성은 예외를 이미 처리했으며 이와 관련해 더이상 아무것도 하지 않아도 된다는 것을 WPF에 전달합니다.
요약
예외 처리는 모든 어플리케이션에서 매우 중요한 부분입니다. 다행히도 WPF와 .NET은 로컬로도, 전역으로도 예외 처리가 굉장히 쉽습니다. 일반적인 경우에는 로컬로 예외 처리를 하고, 폴백 메커니즘으로 전역 예외 처리를 합니다. 왜냐하면 로컬에서 예외 처리를 할 때 더욱 상세하고 특화된 방법으로 문제를 다룰 수 있기 때문입니다.