This article is currently in the process of being translated into Korean (~98% done).
DataGrid with row details
DataGrid 컨트롤을 이용하는 가장 일반적인 사용 시나리오는 자체 Row 아래에 해당 Row에 대한 세부 정보를 표시하는 기능이다. WPF DataGrid 컨트롤은 이 기능을 잘 지원하며, 다행스럽게 사용이 아주 쉽고 간편하다. 아래 예를 보자, 그리고 여러분에게 주어지는 옵션과 어떻게 작동하는지 논의 해보자.
<Window x:Class="WpfTutorialSamples.DataGrid_control.DataGridDetailsSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="DataGridDetailsSample" Height="200" Width="400">
<Grid Margin="10">
<DataGrid Name="dgUsers" AutoGenerateColumns="False">
<DataGrid.Columns>
<DataGridTextColumn Header="Name" Binding="{Binding Name}" />
<DataGridTextColumn Header="Birthday" Binding="{Binding Birthday}" />
</DataGrid.Columns>
<DataGrid.RowDetailsTemplate>
<DataTemplate>
<TextBlock Text="{Binding Details}" Margin="10" />
</DataTemplate>
</DataGrid.RowDetailsTemplate>
</DataGrid>
</Grid>
</Window>
using System;
using System.Collections.Generic;
using System.Windows;
namespace WpfTutorialSamples.DataGrid_control
{
public partial class DataGridDetailsSample : Window
{
public DataGridDetailsSample()
{
InitializeComponent();
List<User> users = new List<User>();
users.Add(new User() { Id = 1, Name = "John Doe", Birthday = new DateTime(1971, 7, 23) });
users.Add(new User() { Id = 2, Name = "Jane Doe", Birthday = new DateTime(1974, 1, 17) });
users.Add(new User() { Id = 3, Name = "Sammy Doe", Birthday = new DateTime(1991, 9, 2) });
dgUsers.ItemsSource = users;
}
}
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public DateTime Birthday { get; set; }
public string Details
{
get
{
return String.Format("{0} was born on {1} and this is a long description of the person.", this.Name, this.Birthday.ToLongDateString());
}
}
}
}
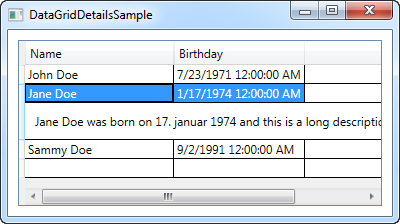
여러분이 보듯이, 사용자 Class에 새로운 porperty를 추가하여 이전 챕터에서 예를 확장했다. Description property(설명 속성): 이것은 세부 Row에 대하여 사용자에 대한 조금의 정보를 간단하게 전달(반환) 할 뿐이다.
마크업에서, 몇개의 열을 정의하고, RowDetailsTemplate로 Row 세부정보를 위한 템플릿을 이용한다. 보는것처럼, 그것은 다른 WPT template처럼 작동한다. 이 Description property에 경우, 그 안에 하나 또는 여러개를 가진 DataTemplate를 이용하고, data 원본에 대한 표준 Binding 속성을 사용한다.
위 결과 스크린샷에서 보는것처럼, 또는 샘플을 직접 실행 했다면, 세부정보가 선택된 Row아래에 표시된다. 다른 Row를 선택하자마자, Row에 대한 세부정보가 보여지고, 이전 선택 Row에 대한 정보는 숨겨진다.
Row 세부정보 보이기 컨트롤
RowDetailsVisibilityMode property를 이용하여, 위에서 이야기한 기능(행동)을 바꿀 수 있다. VisibleWhenSelected 는 디폴드(defaults)이다. 해당 부모 row를 선택할때 단지 세부 정보가 보여지며, 보여지거나(Visible) 보이지 않거나(Collapsed)하게 변경할 수 있다. 보여지기를 원한다면, 모든 세부 Row들은 아래처럼 항상 보이게 할 수 있다.
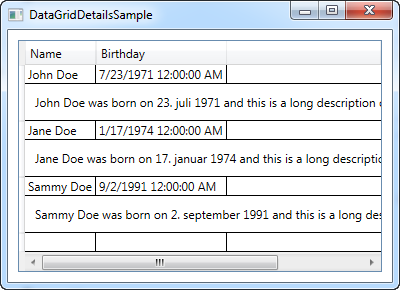
만약 보이지 않게하고 싶다면, 모든 정보가 한번에 안보이게 한다.
더 자세한 내용
첫번째 샘플은 하나의 TextBock 컨트롤을 활요하여, 조금 지루하거나, 단순햇했을 수 있다. 물론, DataTemplate를 이용하여, 우리가 원하는 모든것을 잘 할 수 있다. 그래서 가능성있는 더 좋은 기능을 알기 위해 조금 더 예제를 더 확장 해보기로 한다. 지금, 아래에 보이는 스냅샷이다.
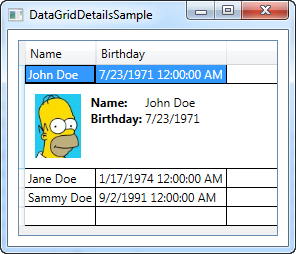
코드 리스트에서 볼 수 있듯이, 더 많은 패널과 또는 컨트롤을 담을 수 있는 패널을 이용한 template를 확장하는 것이다. Gird Panel를 이용하여, 사용자 데이타를 표 모양으로 만들수 있으며, 이미지 컨트롤러는 사용자의 사진을 보여준다.(예제처럼 리소스는 원격 리소스보다는 로칼 리소스를 로드하는 것이 좋다- 째금 게을러서 Jane과 Sammy Doed의 일치하는 이미지를 찾지 못해 미안!!)
<Window x:Class="WpfTutorialSamples.DataGrid_control.DataGridDetailsSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="DataGridDetailsSample" Height="300" Width="300">
<Grid Margin="10">
<DataGrid Name="dgUsers" AutoGenerateColumns="False">
<DataGrid.Columns>
<DataGridTextColumn Header="Name" Binding="{Binding Name}" />
<DataGridTextColumn Header="Birthday" Binding="{Binding Birthday}" />
</DataGrid.Columns>
<DataGrid.RowDetailsTemplate>
<DataTemplate>
<DockPanel Background="GhostWhite">
<Image DockPanel.Dock="Left" Source="{Binding ImageUrl}" Height="64" Margin="10" />
<Grid Margin="0,10">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<TextBlock Text="ID: " FontWeight="Bold" />
<TextBlock Text="{Binding Id}" Grid.Column="1" />
<TextBlock Text="Name: " FontWeight="Bold" Grid.Row="1" />
<TextBlock Text="{Binding Name}" Grid.Column="1" Grid.Row="1" />
<TextBlock Text="Birthday: " FontWeight="Bold" Grid.Row="2" />
<TextBlock Text="{Binding Birthday, StringFormat=d}" Grid.Column="1" Grid.Row="2" />
</Grid>
</DockPanel>
</DataTemplate>
</DataGrid.RowDetailsTemplate>
</DataGrid>
</Grid>
</Window>
using System;
using System.Collections.Generic;
using System.Windows;
namespace WpfTutorialSamples.DataGrid_control
{
public partial class DataGridDetailsSample : Window
{
public DataGridDetailsSample()
{
InitializeComponent();
List<User> users = new List<User>();
users.Add(new User() { Id = 1, Name = "John Doe", Birthday = new DateTime(1971, 7, 23), ImageUrl = "http://www.wpf-tutorial.com/images/misc/john_doe.jpg" });
users.Add(new User() { Id = 2, Name = "Jane Doe", Birthday = new DateTime(1974, 1, 17) });
users.Add(new User() { Id = 3, Name = "Sammy Doe", Birthday = new DateTime(1991, 9, 2) });
dgUsers.ItemsSource = users;
}
}
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public DateTime Birthday { get; set; }
public string ImageUrl { get; set; }
}
}
요약
이 튜토리얼의 예에서 볼 수 있듯이, DataGrid row는 세부정보를 표시하는 기능은 아주 유용하며, WPF DataGrid는 쉽고, 커스트마이징하기 좋다.