This article is currently in the process of being translated into Hindi (~57% done).
Command-line parameters in WPF
Command-line पैरामीटर एक ऐसी तकनीक है जहां आप पैरामीटर के सेट को ऐपलिकेशन में पास कर सकते हैं जिसे आप शुरू करना चाहते हैं, किसी तरह इसे प्रभावित करने के लिए। सबसे आम उदाहरण ऐपलिकेशन को एक विशिष्ट फ़ाइल के साथ खुला बनाना है, उदा। एक संपादक(editor) में। आप इसे विंडोज के अंतर्निहित नोटपैड एप्लिकेशन के साथ चलाकर (स्टार्ट मेनू से रन चुनें या [विंडोज की-R]) दबाकर देख सकते हैं:
notepad.exe c:\Windows\win.ini
इससे नोटपैड(Notepad) खुलेगा win.ini फ़ाइल के साथ (आपको अपने सिस्टम से मिलान करने के लिए पथ को समायोजित करना पड़ सकता है)। नोटपैड बस एक या कई पैरामीटर की तलाश करता है और फिर उनका उपयोग करता है और आपका ऐपलिकेशन भी वही कर सकता है!
Command-line पैरामीटर स्टार्टअप इवेंट(Startup event) के माध्यम से आपके WPF एप्लिकेशन को पास किए जाते हैं, जिसे हमने App.xaml लेख में सब्सक्राइब किया था। हम इस उदाहरण में भी ऐसा ही करेंगे और फिर मैथड आरग्यूमेंट्स(method arguments) के माध्यम से पास किए गए वैल्यू(value) का उपयोग करेंगे। सबसे पहले, App.xaml फ़ाइल:
<Application x:Class="WpfTutorialSamples.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Startup="Application_Startup">
<Application.Resources></Application.Resources>
</Application>
हमें बस यहां StartupUri प्रॉपर्टी की जगह Startup इवेंट को सब्सक्राइब करना है। इवेंट को फिर App.xaml.cs में लागू किया गया है:
using System;
using System.Collections.Generic;
using System.Windows;
namespace WpfTutorialSamples
{
public partial class App : Application
{
private void Application_Startup(object sender, StartupEventArgs e)
{
MainWindow wnd = new MainWindow();
if(e.Args.Length == 1)
MessageBox.Show("Now opening file: \n\n" + e.Args[0]);
wnd.Show();
}
}
}
StartupEventArgs जो हम यहां उपयोग करते हैं, यह 'e' नाम के साथ 'Application Startup' इवेंट में पारित किया गया है। इसके पास Args प्रॉपर्टी है, जो स्ट्रिंग्स की एक सरणी(Array) है। कमांड-लाइन पैरामीटरों को रिक्त स्थान द्वारा अलग किया जाता है, जब तक कि रिक्त स्थान एक उद्धृत स्ट्रिंग(Quoted String) के अंदर न हो।
command-line parameter परीक्षण
If you run the above example, nothing will happen, because no command-line parameters have been specified. Fortunately, Visual Studio makes it easy to test this in your application. From the Project menu select "[Project name] properties" and then go to the Debug tab, where you can define a command-line parameter. It should look something like this:
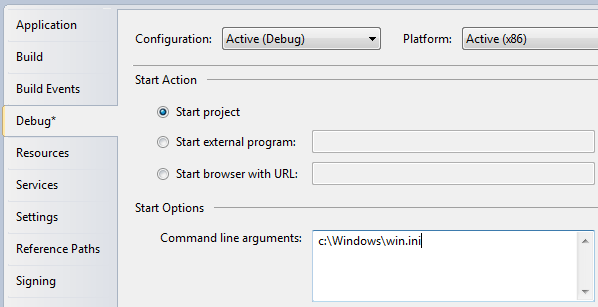
Try running the application and you will see it respond to your parameter.
Of course, the message isn't terribly useful. Instead you might want to either pass it to the constructor of your main window or call a public open method on it, like this:
using System;
using System.Collections.Generic;
using System.Windows;
namespace WpfTutorialSamples
{
public partial class App : Application
{
private void Application_Startup(object sender, StartupEventArgs e)
{
MainWindow wnd = new MainWindow();
// The OpenFile() method is just an example of what you could do with the
// parameter. The method should be declared on your MainWindow class, where
// you could use a range of methods to process the passed file path
if(e.Args.Length == 1)
wnd.OpenFile(e.Args[0]);
wnd.Show();
}
}
}
Command-line possibilities
In this example, we test if there is exactly one argument and if so, we use it as a filename. In a real world example, you might collect several arguments and even use them for options, e.g. toggling a certain feature on or off. You would do that by looping through the entire list of arguments passed while collecting the information you need to proceed, but that's beyond the scope of this article.