This article is currently in the process of being translated into Korean (~79% done).
ListView, data binding and ItemTemplate
이전 글에서, 우리는 XAML code를 통해 ListView control을 수동으로 채워보았습니다. 하지만 WPF에선 데이터를 채우는 과정은 보통 data binding으로 이뤄집니다. data binding에 대한 개념은 이 tutorial의 다른 part에 자세하게 설명돼있기 때문에 단순히 말하자면 layout에서 데이터를 분리하는 것 입니다. 한번 ListView에 일련의 data를 바인딩 해보겠습니다.
<Window x:Class="WpfTutorialSamples.ListView_control.ListViewDataBindingSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="ListViewDataBindingSample" Height="300" Width="300">
<Grid>
<ListView Margin="10" Name="lvDataBinding"></ListView>
</Grid>
</Window>
using System;
using System.Collections.Generic;
using System.Windows;
namespace WpfTutorialSamples.ListView_control
{
public partial class ListViewDataBindingSample : Window
{
public ListViewDataBindingSample()
{
InitializeComponent();
List<User> items = new List<User>();
items.Add(new User() { Name = "John Doe", Age = 42 });
items.Add(new User() { Name = "Jane Doe", Age = 39 });
items.Add(new User() { Name = "Sammy Doe", Age = 13 });
lvDataBinding.ItemsSource = items;
}
}
public class User
{
public string Name { get; set; }
public int Age { get; set; }
}
}
우리는 각각 이름과 나이를 가진 user로 구성된 User objects들로 list로 채웠습니다. 우리가 ListView의 ItemsSource property에 list를 할당하자 마자 data binding이 자동으로 수행되지만 결과는 약간 실망스럽습니다.
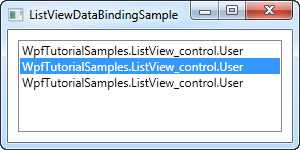
각 user는 ListView 내에서 그들의 type 명으로 표시됩니다. 이는 당신이 당신의 data를 어떻게 표시되게 할 지에 대한 단서를 .NET에 제공하지 않았기 때문에 나타난 현상입니다. 그래서 .NET은 그저 각 object에 대해 ToString() 메소드를 수행했고 이를 사용하여 item을 표시했습니다.
We can use that to our advantage and override the ToString() method, to get a more meaningful output. Try replacing the User class with this version:
public class User
{
public string Name { get; set; }
public int Age { get; set; }
public override string ToString()
{
return this.Name + ", " + this.Age + " years old";
}
}
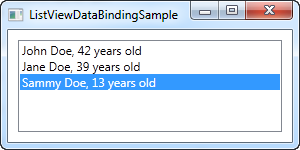
이건 훨씬 더 사용자 친화적 표기이며 몇몇 경우에 괜찮을 방안입니다. 하지만 단순 문자열에 의존하는 건 유연하지 않습니다. 텍스트의 일부를 굵게하거나 다른 색깔로 표시하고 싶나요? 이미지를 표시하고 싶나요? 운좋게도 WPF는 templates를 사용해 매우 간단하게 해결책을 제시합니다.
ItemTemplate를 가진 ListView
WPF는 모두 template으로 이뤄졌기 때문에 ListView에 대한 data template을 작성하는 것은 매우 쉽습니다. 이 예제에서 우리는 단지 당신에게 이것이 WPF ListView를 얼마나 유연하게 해주는지 보여주기 위해 각 항목에 여러가지 맞춤 서식을 작성하겠습니다.
<Window x:Class="WpfTutorialSamples.ListView_control.ListViewItemTemplateSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="ListViewItemTemplateSample" Height="150" Width="350">
<Grid>
<ListView Margin="10" Name="lvDataBinding">
<ListView.ItemTemplate>
<DataTemplate>
<WrapPanel>
<TextBlock Text="Name: " />
<TextBlock Text="{Binding Name}" FontWeight="Bold" />
<TextBlock Text=", " />
<TextBlock Text="Age: " />
<TextBlock Text="{Binding Age}" FontWeight="Bold" />
<TextBlock Text=" (" />
<TextBlock Text="{Binding Mail}" TextDecorations="Underline" Foreground="Blue" Cursor="Hand" />
<TextBlock Text=")" />
</WrapPanel>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</Grid>
</Window>
using System;
using System.Collections.Generic;
using System.Windows;
namespace WpfTutorialSamples.ListView_control
{
public partial class ListViewItemTemplateSample : Window
{
public ListViewItemTemplateSample()
{
InitializeComponent();
List<User> items = new List<User>();
items.Add(new User() { Name = "John Doe", Age = 42, Mail = "john@doe-family.com" });
items.Add(new User() { Name = "Jane Doe", Age = 39, Mail = "jane@doe-family.com" });
items.Add(new User() { Name = "Sammy Doe", Age = 13, Mail = "sammy.doe@gmail.com" });
lvDataBinding.ItemsSource = items;
}
}
public class User
{
public string Name { get; set; }
public int Age { get; set; }
public string Mail { get; set; }
}
}
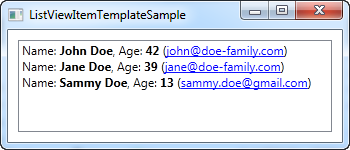
우리는 여러 TextBlock 컨트롤을 사용하여 각 항목을 만들고 텍스트의 일부를 굵게 표시했습니다. 이메일 주소에는 하이퍼링크처럼 동작하도록 만들기 위해 밑줄을 긋고 파란색으로 설정 후 마우스 커서 모양을 바꿨습니다.
요약
ItemTemplate과 data binding을 사용함으로써 우리는 꽤 멋진 ListView control을 만들었습니다. 하지만 여전히 이것은 ListBox와 흡사합니다. ListView가 사용되는 가장 흔한 경우는 columns(때로는 details view로 언급되는)를 갖는 것입니다. WPF에는 이를 처리하기 위한 내장 view class를 가지고 있으며 다음 chapter에서 소개합니다.