This article is currently in the process of being translated into Turkish (~74% done).
FlowDocument Kontrolünü Arkaplan Kodunda Üretmek
Şimdiye kadar FlowDocument'leri hep direkt XAML içinde tanımladık. XAML tüm internette sayfaları üretmekte kullanılan HTML kodlarına benzediği için bir dökümanı göstermek için mantıklı geliyor. Buna rağmen bu FlowDocument kontrollerini arkaplan kodundan üretemeyeceğiniz anlamına gelmez - tabi ki yapabilirsiniz, her eleman bir sınıf olarak ifade edildiği için bu sınıftan bir nesne üreterek klasik C# programlarında yaptığımızı uygulayabiliriz.
As a bare minimum example, here's our "Hello, world!" example from one of the first articles, created from Code-behind instead of XAML:
<Window x:Class="WpfTutorialSamples.Rich_text_controls.CodeBehindFlowDocumentSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="CodeBehindFlowDocumentSample" Height="200" Width="300">
<Grid>
<FlowDocumentScrollViewer Name="fdViewer" />
</Grid>
</Window>
using System;
using System.Windows;
using System.Windows.Documents;
using System.Windows.Media;
namespace WpfTutorialSamples.Rich_text_controls
{
public partial class CodeBehindFlowDocumentSample : Window
{
public CodeBehindFlowDocumentSample()
{
InitializeComponent();
FlowDocument doc = new FlowDocument();
Paragraph p = new Paragraph(new Run("Hello, world!"));
p.FontSize = 36;
doc.Blocks.Add(p);
p = new Paragraph(new Run("The ultimate programming greeting!"));
p.FontSize = 14;
p.FontStyle = FontStyles.Italic;
p.TextAlignment = TextAlignment.Left;
p.Foreground = Brushes.Gray;
doc.Blocks.Add(p);
fdViewer.Document = doc;
}
}
}
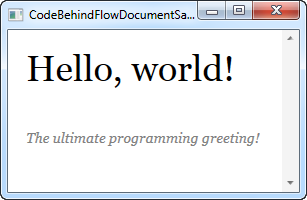
Aynı işlevi yerine getirebilecek az miktardaki XAML koduna karşılık pekte etkileyici değil:
<FlowDocument>
<Paragraph FontSize="36">Hello, world!</Paragraph>
<Paragraph FontStyle="Italic" TextAlignment="Left" FontSize="14" Foreground="Gray">The ultimate programming greeting!</Paragraph>
</FlowDocument>
Uzun kod satırlarını bir kenara bırakırsak, bazı zamanlarda işleri Code-behind üzerinden yürütmek daha makul olabilir ve yazıda gösterildiği gibi bu mümkündür.