This article has been localized into Czech by the community.
Použití DataContext
Vlastnost DataContext je výchozím zdrojem vazeb, pokud výslovně nedeklarujete jiný zdroj, jak tomu bylo v předchozí kapitole s vlastností ElementName. Je definován ve třídě FrameworkElement, kterou dědí většina ovládacích prvků uživatelského rozhraní, včetně okna WPF. Jednoduše řečeno, umožňuje blíže určit základ vašich vazeb.
Neexistuje výchozí zdroj pro vlastnost DataContext (na začátku má jednoduše hodnotu null), ale protože je DataContext zděděn přes hierarchii ovládacích prvků, můžete nastavit DataContext pro samotné okno formuláře a pak jej použít ve všech podřízených ovládacích prvcích. Zkusme si to ilustrovat na jednoduchém příkladu:
<Window x:Class="WpfTutorialSamples.DataBinding.DataContextSample"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="DataContextSample" Height="130" Width="280">
<StackPanel Margin="15">
<WrapPanel>
<TextBlock Text="Window title: " />
<TextBox Text="{Binding Title, UpdateSourceTrigger=PropertyChanged}" Width="150" />
</WrapPanel>
<WrapPanel Margin="0,10,0,0">
<TextBlock Text="Window dimensions: " />
<TextBox Text="{Binding Width}" Width="50" />
<TextBlock Text=" x " />
<TextBox Text="{Binding Height}" Width="50" />
</WrapPanel>
</StackPanel>
</Window>
using System;
using System.Windows;
namespace WpfTutorialSamples.DataBinding
{
public partial class DataContextSample : Window
{
public DataContextSample()
{
InitializeComponent();
this.DataContext = this;
}
}
}
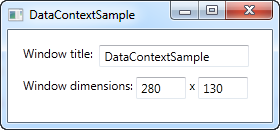
V tomto příkladu kód na pozadí přidá pouze jeden zajímavý řádek: po standardním volání InitalizeComponent () přiřadíme odkaz "this" k vlastnosti DataContext, která v podstatě pouze sděluje Oknu (Window), že chceme, aby bylo samotné datovým kontextem.
V XAML využíváme tohoto faktu k navázání na několik vlastností okna, včetně Title (název), Width (šířka) a Height (výška). Jelikož okno má DataContext, který je předáván dolů k dětským ovládacím prvkům (potomkům), nemusíme definovat zdroj pro každou z vazeb - používáme hodnoty, jako by byly globálně dostupné.
Zkuste spustit příklad a změnit velikost okna - uvidíte, že změny rozměrů jsou okamžitě zobrazeny v textových polích. Můžete také zkusit napsat jiný název do prvního textového pole, ale možná budete překvapeni, že tato změna není okamžitě zobrazena. Místo toho musíte přesunout fokus na jiný ovládací prvek, než je změna aplikována. Proč? No, to bude předmětem příští kapitoly.
Shrnutí
Použití vlastnosti DataContext je jako nastavení základu pro všechny vazby (bindingy) skrze hierarchii ovládacích prvků. Ušetří vám to práci s ručním definováním zdroje pro každou vazbu, a jakmile skutečně začnete datové vazby (data bindingy) používat, určitě oceníte ušetřený čas a psaní.
To ale neznamená, že musíte pro všechny ovládací prvky v rámci okna používat stejný DataContext. Jelikož každý ovládací prvek má svou vlastní vlastnost DataContext, můžete snadno přerušit řetězec dědičnosti a přepsat DataContext novou hodnotou. To vám umožní dělat věci jako mít globální DataContext na okně a pak mít lokálnější a specifičtější DataContext například na panelu, který drží samostatný formulář nebo něco podobného.